Yii 2.0 Writing Components
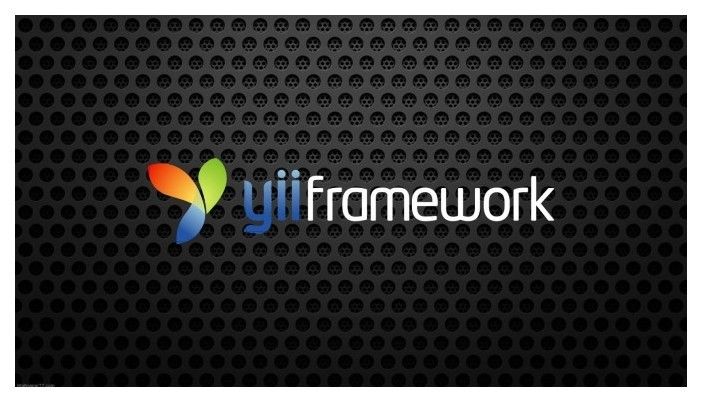
In this post I want to teach you how to write components in Yii 2.0 Why do we need to write components? As an example, I can say, imagine you want to write a function and call it wherever you need within your website.
In this post I want to teach you how to write components in Yii 2.0
Why do we need to write components?
As an example, I can say, imagine you want to write a function and call it wherever you need within your website. Well, in this case you need to write a component. Or imagine you need to write several functions which you can call wherever you want within your project. These can be example of why we need to know how to write components in Yii 2.0.
How to add a component to Yii 2.0?
Now that you know how important it is to know how to add components, let's get started on the process of adding components.
In your projects, there should be a folder named components. If you cannot find it, add it to your projects. In this folder add your php file. As an example we want a file full of functions. We name it PublicFunctions.php
Now, in our file we should start like this:
<?php namespace app\components; use Yii; use yii\base\Component; use yii\base\InvalidConfigException; class PublicFunctions extends Component { }
This is our Component. Now we can add as many functions as we want the this class. As an example:
<?php namespace app\components; use Yii; use yii\base\Component; use yii\base\InvalidConfigException; class PublicFunctions extends Component { public function getAll($model, $condition = null) { $model = "\\app\\models\\" . $model; return $model::find()->where($condition)->all(); } }
How to tell Yii that we have added a component to our project?
Well, this is simple. Open web.php file which is your config file (you can find it in config folder) and add the following line to $config array:
'publicFunctions' => [ 'class' => 'app\components\PublicFunctions' ],
So in the code above, we have told Yii that we have a component. Its name is publicFunctions and the path (as you can see class) to it is app\components\PublicFunctions. Now Yii knows about our component file.
How can we use the function we have written in our component?
This is simple too! To call your component, simple use Yii::$app->componentName->componentFunction
In our example, we can call getAll() like this:
Yii::$app->publicFunctions->getAll('Person');
Now you have learnt how to write a component in Yii 2.0