Yii 2.0 find (SELECT)
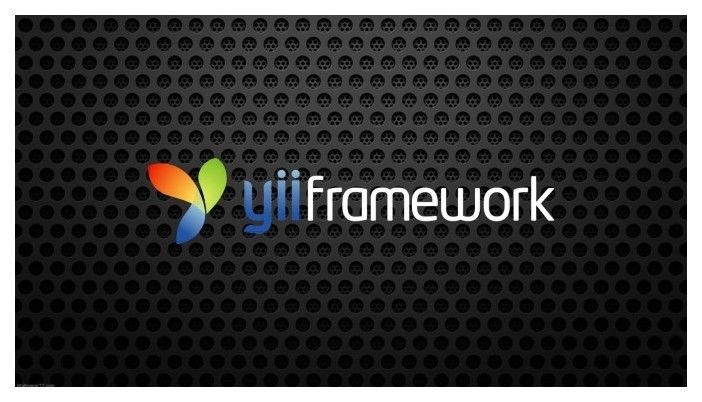
In this post I want to talk about selecting (fetching) records from database. NOTE: When you want to download Yii 2.0, you can see that Yii 2.0 is not backward compatible. This means, some functions and syntaxes have changed.
In this post I want to talk about selecting (fetching) records from database.
NOTE: When you want to download Yii 2.0, you can see that Yii 2.0 is not backward compatible. This means, some functions and syntaxes have changed.So, if you have developed a website using Yii 1.x, you cannot easily migrate it to Yii 2.0. You have to revise your codes. By revising, I mean reading your codes again and changing some of your previously written codes. One of the parts you have to change is the functions you have written which fetch records from database.
Let's start with an example. Imagine we have one table which is called ‘Person‘. ‘Person‘ attributes are, ‘pid, name, type‘ and our primary key is ‘pid‘.
If we want to fetch all records in our table, the right SQL syntax would be:
"SELECT * FROM Person"
I assume that you know what MODELS are. So, we have created our Person Model. When we want to write our query, we have to mention the name of our model, in this case it's Person. To use MODELS we have to use models library which is \app\models. So our query would be:
'$people = \app\models\Person::find()->all();'
With this query we fetch all records in our database. If you want to add conditions to your query, you can simply add where() to it. As an example, we want to fetch all records which their type is user. Our SQL query would be:
'SELECT * FROM Person WHERE type="user"'
This will change to:
'$people = \app\models\Person::find()->where(['type'=>'user'])->all();'
If you want to fetch only one record, instead of all() you can use one(). As an example we want to fetch the record which its pid is 1.
Our SQL query would be:
'SELECT * FROM Person WHERE pid=1'
This will change to:
'$people = \app\models\Person::find()->where(['pid'=>1])->one();'
You can also use groupBy() and orderBy() as SQL GROUP BY and ORDER BY, in the same way you used where(). You can write a string as the condition for where and append the parameters to it. There are other types of where which one of them is andWhere() and the other is orWhere(). You can use them together with where() in queries which some of your conditions are taking an array as their condition (like above) and the rest are taking a string as their condition.
As an example:
'$people = \app\models\Person::find()->where(['pid'=>1])->andWhere("type = user")->one();'
This query can be written in another way too:
'$people = \app\models\Person::find()->where(['pid'=>1,'type'=>'user'])->one();'