Yii 2.0 Ajax
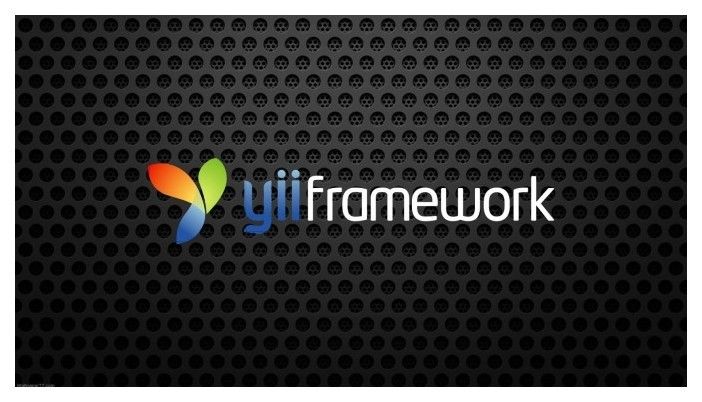
In this post I want to talk about using ajax in Yii 2.0. I assume you all know what ajax is and how to use an ajax request.
In this post I want to talk about using ajax in Yii 2.0.
I assume you all know what ajax is and how to use an ajax request.
A quick review of how to send an ajax request:
Imagine in our HTML file we have a link with its class attribute set to delete-pic. So it would look like the following:
<a class="delete-pic" fileId="<?php echo $file->id; ?>">Delete Pic</a>
Now, we want to delete our picture using its id and ajax. So in our javascript we should do something like the following (remember: baseUrl is defined in our header in a javascript tag) (remember: send CSRF if you have turned it on!)
$('.delete-pic').click(function (e) { clicked = this; fid = $(clicked).attr('fileId'); $.ajax({ url: baseUrl + "/image/delete", type: "post", data: {'id': fid, _csrf: yii.getCsrfToken()}, dataType: "JSON", success: function (response) { if (response.status == "done") { alert('Done!'); } else if (response.status == "failed") { alert('Failed to delete the Image'); } } }); e.preventDefault(); });
How should we handle Ajax requests in Yii 2.0?
As you all know, when using ajax, you have to send your request to a url. In Yii 2.0 urls are created with the following pattern: <controller>/<action>
So if you want to delete a picture using ajax, first you have to have a controller and an action for that. Imagine the controller is called ImageController and the action is actionDelete. So you have to send your request to basePath/image/delete. (basePath is your website's basepath, such as www.example.com) So as we said, your ajax request and the values you want to use in your request should be sent to basePath/image/delete. We don't want people to see whatever is happening in our action directly by using its url. So if someone enters basePath/image/delete in the url, we want him to see a 404 error. So you should start your action like the following:
public function actionDelete() { header('Content-type: application/json'); if (!Yii::$app->request->isAjax){ throw new \yii\web\HttpException(404, 'The specified post cannot be found.'); } Yii::$app->end(); }
Now, after if, we have to delete our image. So it would look like the following (We have sent the id of the image we want to delete in an array using post method):
public function actionDelete() { header('Content-type: application/json'); if (!Yii::$app->request->isAjax){ throw new \yii\web\HttpException(404, 'The specified post cannot be found.'); } $file=\app\models\File::find()->where(['id']=>$_POST['id'])->one(); if($file->delete()){ echo json_encode(['status'=>'done']); } else{ echo json_encode(['status'=>'failed']); } Yii::$app->end(); }
The code above find's the image stored in the database, and tries to delete it. If this happens successfully, we return to our javascript and we send a parameter called status to it with the value of done. If not, we send status to it with the value of failed. And we can handle the rest in our javascript as usual.