MongoDB sub-documents (Basics)
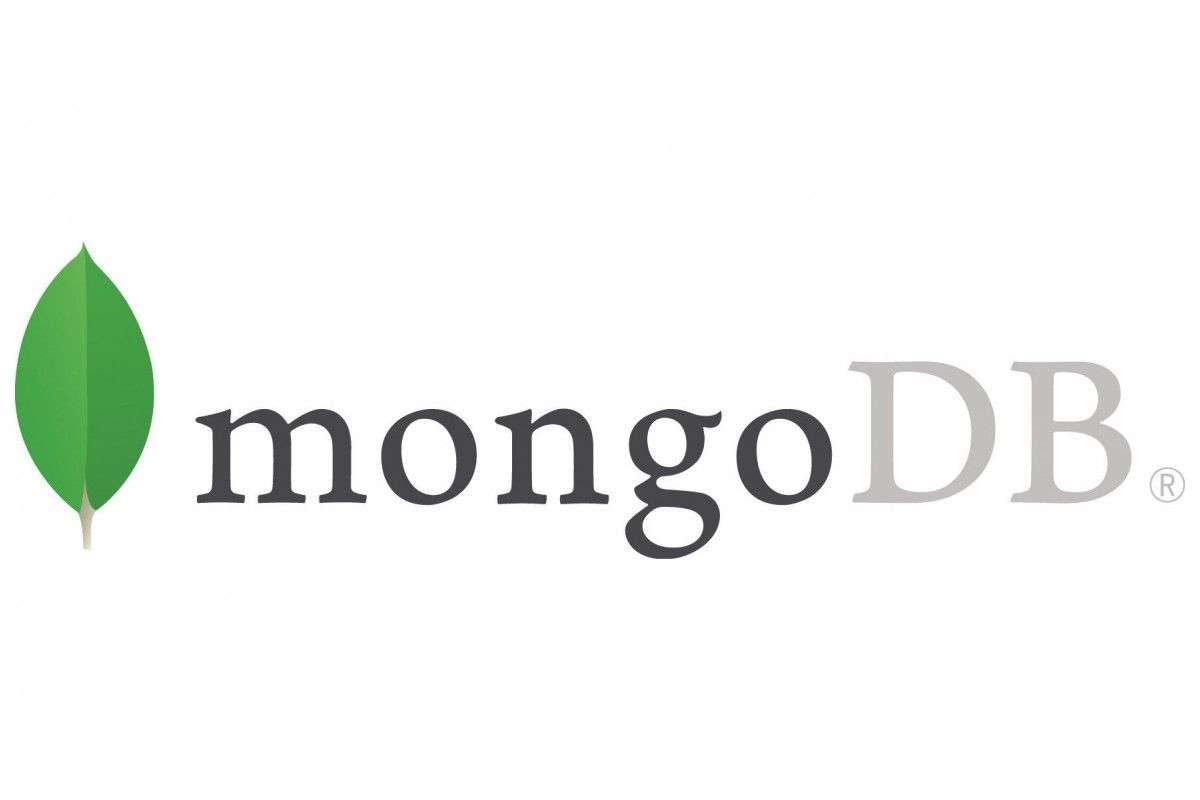
As we all know, MongoDB is a document-oriented database which is a NoSQL database. One of the great features of MongoDB is supporting sub-documents. In this post I am going to talk about some basic things about using sub-documents that usually most Relational Database Designers and Developers (like me) find challenging.
As we all know, MongoDB is a document-oriented database which is a NoSQL database. One of the great features of MongoDB is supporting sub-documents. In this post I am going to talk about some basic things about using sub-documents that usually most Relational Database Designers and Developers (like me) find challenging.
Sub-Documents
I assume you know what collections and documents are. In MongoDB a document is represented as a JSON document. Imagine we want to store a user, his/her address, and phone numbers. As we all know, when working with RDBs we can store the user in a table called 'user', his/her address in a table called 'address' with a foreign key that refers to the user_id, and related phone numbers in another table called 'phone_numbers' with a foreign key that refers to the address_id. If we want to do the same thing in MongoDB we have to have a 'users' collection, 'addresses' collection, and a 'phone_numbers' collections and each document in these collections will look something like:
users:
{ "_id": ObjectID("5ae41b5965a9d4390d1ac3ef"), "name": "John Doe" }
addresses:
{ "_id": ObjectId("5ae42b5965a9d4390d1ac5ef"), "address": "NO.4, First Street, London, UK", "user_id": ObjectID("5ae41b5965a9d4390d1ac3ef") }
phone_numbers:
{ "_id": ObjectID("5ae41b5965a9d4390d1ac6ef"), "number": "555-555-555", "address_id": ObjectID("5ae42b5965a9d4390d1ac5ef") } { "_id": ObjectID("5ae41b5965a9d4390d1ac8ef"), "number": "444-444-444", "address_id": ObjectID("5ae42b5965a9d4390d1ac5ef") }
So we have one user with an address and two phone numbers. However, with the use of sub-documents, which basically are documents inside other documents, we can have the following document in a single collection called 'users' collection:
{ "_id": ObjectID("5ae41b5965a9d4390d1ac3ef"), "name": "John Doe", "address_sd": { "address": "NO.4, First Street, London, UK", "phone_numbers": [ "555-555-555", "444-444-444" ] } }
So as you can see we have a sub-document named address_sd which contains an address and an array of phone numbers, called phone_numbers. So basically we have denormalized our design.
Should we always use sub-documents?
Most of us think because MongoDB supports sub-documents, we should always think of using them. However, this is not always true. So when should we avoid using them? I have struggled for a long time and read some blog posts on MongoDB website and could understand when not to use them. I want to tell you two conditions that you have to consider when using sub-documents:
- When you want to have direct access to the sub-document regardless of the parent document you certainly must avoid using sub-documents. Imagine having a user who has written several blog posts. Instead of storing his posts inside his user document, you can have another collection called 'blog_posts' and store the post in this collection, just like a Relational Database.
- Also, when you are sure that as mentioned above the sub-document does not need to be accessed directly, if there are a lot of sub-documents that should be embedded in our document, avoid using sub-documents. You may ask, why. Well, by the time of writing this post, MongoDB has a 16 Megabytes restriction on document size, which means size of each document in a collection cannot exceed 16 Megabytes.
So always check the conditions above to decide whether or not you should use sub-documents.