MongoDB Indexes - Part 2
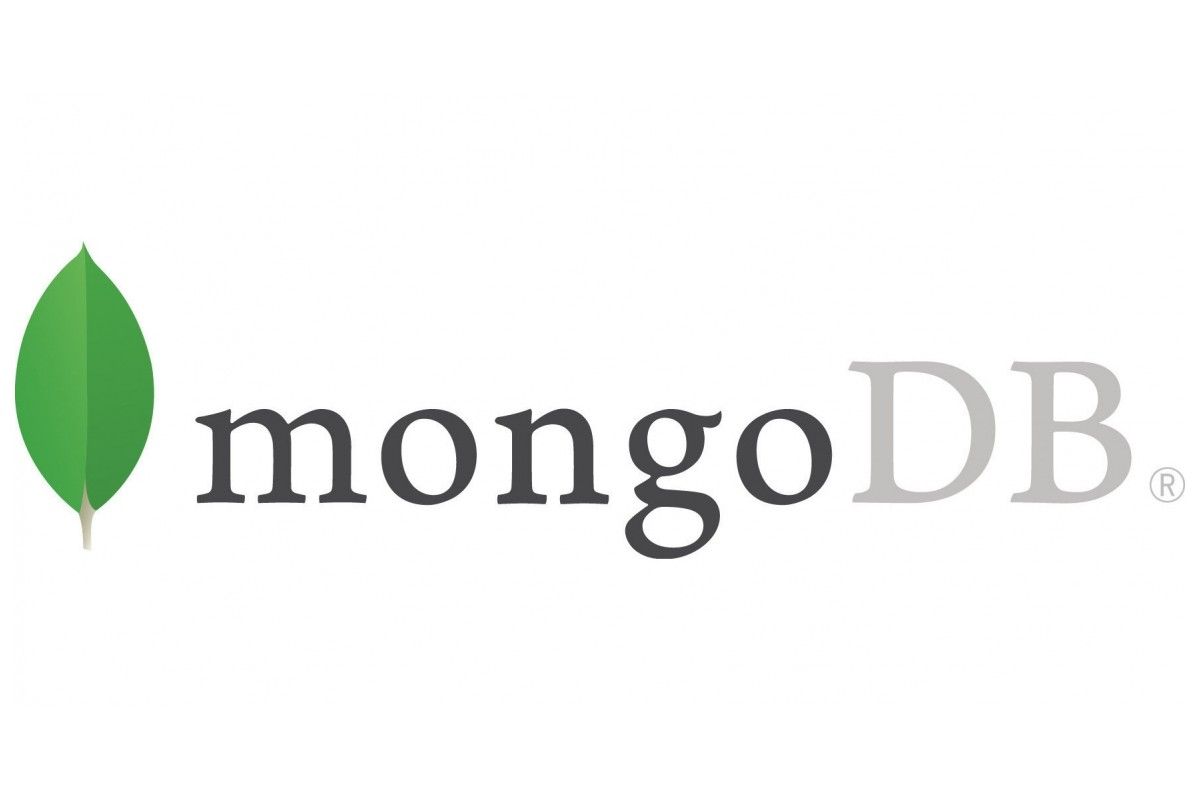
This is part two of a two-part series on MongoDB indexes. In my previous post, I talked about what indexes are, how they can help us, and some types of indexes (Single Field Index, Compound Index, Partial Index, and Sparse Index). In this post, I will try to explain two other types of MongoDB (Multikey Index and Text Index).
This is part two of a two-part series on MongoDB indexes. In my previous post, I talked about what indexes are, how they can help us, and some types of indexes (Single Field Index, Compound Index, Partial Index, and Sparse Index). In this post, I will try to explain two other types of MongoDB Indexes (Multikey Index and Text Index).
Multikey Index
This type of index is used to index array fields (fields containing arrays) and index each element in the array. These fields can contain both scalar and nested document values. To create this type of index, we can use the same command we used for a single field index.
db.phoneBook.createIndex( { cities: -1 } )
The above code tells MongoDB to create this index in a descending order. To have an ascending index, we can change -1 to 1. As you can see, we didn’t mentioned anything about the index being a multikey index. MongoDB will automatically create a multikey index if the field contains array values.
When creating multikey indexes, we must remember that there are some limitations when it comes to creating compound indexes. We cannot create a compound index in which more than one field is an array field. Imagine the following is an example document in our collection:
{ name: “Hirad Daneshvar”, cities: [“A”, “B”], numbers: [ {type: “mobile”, number: “12345”}, {type: “home”, number: “78945”} ] }
We cannot create a compound index on cities and numbers since only one of the fields in our compound index can be an array field. Also, if we have a compound index, we cannot insert a document that violates the previous rule. Imagine we have created an index on name and cities. Since only one of these documents is an array field, our compound index will be created successfully. Imagine we want to insert the following document:
{ name: [“Hirad”, “Daneshvar”], cities: [“A”, “B”], numbers: [ {type: “mobile”, number: “12345”}, {type: “home”, number: “78945”} ] }
MongoDB doesn’t let us insert the above document since both name and cities are array fields and it violates the ruled I mentioned earlier.
Text Index
When we want to look for a text in our database and we only know part of the text there are several options. One option is to use regular expressions. However, they are not very accurate. MongoDB supports text indexes for text queries. The field we want to create a text index on must contain string or it must be an array of string elements. The following will create a text index on name:
db.phoneBook.createIndex( { cities: “text” } )
We can also create compound text indexes:
db.phoneBook.createIndex( { firstName: “text”, lastName:”text” } )
You can also specify language when creating this type of index. MongoDB supports various languages for text searches which help understanding stop words in that language.
The following search query looks for those documents with the text index containing the word tom:
db.phoneBook.find( { $text: { $search: “tom” } } )
We can also limit our query by adding additional conditions:
db.phoneBook.find( { status: “active”, $text: { $search: “tom” } } )
Text index stores an index for every word in the string. Hyphens are like spaces, so if a word considers hyphens, think of it as more than one word, as a result more than one index will be stored for that word. As an example, for the word “T-Shirt” it stores one index for “T” and one for “Shirt”. So, be careful when using text indexes and try using other strategies since this approach can take up a huge amount of RAM.